NS3 VIDEO STREAMING PROJECTS is the act of transmitting compressed (typically) video across a private or public network (like the Internet, a LAN, satellite, or cable television) to be uncompressed and played on a computing device (such as a TV, smart phone or computer).
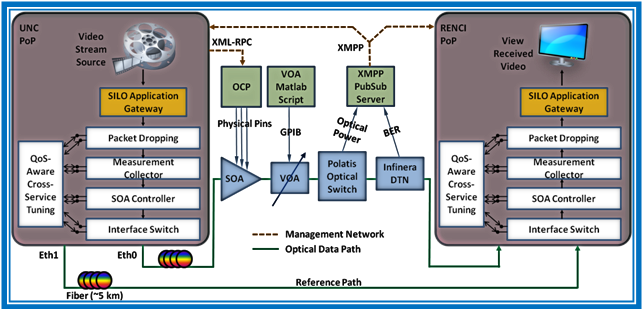
Steps involved in NS3 video streaming projects:
- Capture.
- Conversion.
- Preprocess.
- Encode.
- Transmission.
- Decode.
- Presentation.
Factors considered during streaming videos:
- Video aspect ratio.
- Quality of original source video.
- Streaming bit rate.
- Input video resolution.
- Configuration of the encoder.
- Content of the source including the amount of motion and proper contrast.
- Efficiency of the encoder.
Sample code for NS3 Video Streaming Projects:
Source code for video streaming concepts.
int main(int argc, char **argv) {
uint32_t size = 5;
double totalTime = 50;
uint32_t run = 1;
uint32_t seed = 3945244811;
double xmax = 80;
double ymax = 80;
double pulltime = 15;//in ms
uint32_t pullmax = 1;
bool helloactive = true;
double hellotime = 4;
uint32_t helloloss = 1;
bool pullactive = false;
uint32_t routing = 0;
double PLref = 30.0;
double PLexp = 2.0;
double TxStart = 18.0;
double TxEnd = 18.0;
uint32_t TxLevels = 1;
double EnergyDet= -95.0;
CommandLine cmd;
cmd.AddValue (“size”, “Number of nodes.”, size);
cmd.AddValue (“time”, “Simulation time, s.”, totalTime);
cmd.AddValue (“run”, “Run Identifier”, run);
cmd.AddValue (“seed”, “Seed “, seed);
cmd.AddValue (“PLref”, “Reference path loss dB.”, PLref);
cmd.AddValue (“PLexp”, “Path loss exponent.”, PLexp);
cmd.AddValue (“TxStart”, “Transmission power start dBm.”, TxStart);
cmd.AddValue (“TxEnd”, “Transmission power end dBm.”, TxEnd);
cmd.AddValue (“TxLevels”, “Transmission power levels.”, TxLevels);
cmd.AddValue (“EnergyDet”, “Energy detection threshold dBm.”, EnergyDet);
cmd.AddValue (“CCAMode1”, “CCA mode 1 threshold dBm.”, CCAMode1);
cmd.AddValue (“xmax”, “Grid X max”, xmax);
cmd.AddValue (“ymax”, “Grid Y max”, ymax);
cmd.AddValue (“routing”, “Unicast Routing Protocol (1 – AODV) “, routing);
cmd.AddValue (“hellotime”, “Hello time”, hellotime);
cmd.AddValue (“helloloss”, “Max number of hello loss to be removed from neighborhood”, helloloss);
cmd.AddValue (“helloactive”, “Hello activation”, helloactive);
cmd.AddValue (“pullactive”, “Pull activation allowed”, pullactive);
cmd.AddValue (“pullmax”, “Max number of pull allowed per chunk”, pullmax);
cmd.AddValue (“pulltime”, “Time between pull in ms (e.g., 100ms = 0.100s)”, pulltime);
cmd.AddValue (“v”, “Verbose”, verbose);
cmd.Parse(argc, argv);
SeedManager::SetRun (run);
SeedManager::SetSeed (seed);
Config::SetDefault (“ns3::WifiRemoteStationManager::FragmentationThreshold”, StringValue(“2346”));
Config::SetDefault (“ns3::WifiRemoteStationManager::RtsCtsThreshold”, StringValue(“2346”));
Config::SetDefault (“ns3::LogDistancePropagationLossModel::ReferenceLoss”, DoubleValue(PLref));
Config::SetDefault (“ns3::LogDistancePropagationLossModel::Exponent”, DoubleValue(PLexp));
Config::SetDefault (“ns3::VideoPushApplication::PullActive”, BooleanValue(pullactive));
Config::SetDefault (“ns3::VideoPushApplication::PullTime”, TimeValue(Time::FromDouble(pulltime,Time::MS)));
Config::SetDefault (“ns3::VideoPushApplication::PullMax”, UintegerValue(pullmax));
Config::SetDefault (“ns3::VideoPushApplication::HelloActive”, BooleanValue(helloactive));
Config::SetDefault (“ns3::VideoPushApplication::HelloTime”, TimeValue(Time::FromDouble(hellotime,Time::S)));
Config::SetDefault (“ns3::VideoPushApplication::HelloLoss”, UintegerValue(helloloss));
Config::SetDefault (“ns3::VideoPushApplication::Source”, Ipv4AddressValue(Ipv4Address(“10.0.0.1”)));
Config::SetDefault (“ns3::Ipv4L3Protocol::DefaultTtl”, UintegerValue (1)); //avoid to forward broadcast packets
Config::SetDefault (“ns3::Ipv4::IpForward”, BooleanValue (false));
Config::SetDefault (“ns3::YansWifiPhy::TxGain”,DoubleValue(0.0));
Config::SetDefault (“ns3::YansWifiPhy::RxGain”,DoubleValue(0.0));
Config::SetDefault (“ns3::YansWifiPhy::TxPowerStart”,DoubleValue(TxStart));
Config::SetDefault (“ns3::YansWifiPhy::TxPowerEnd”,DoubleValue(TxEnd));
Config::SetDefault (“ns3::YansWifiPhy::TxPowerLevels”,UintegerValue(TxLevels));
Config::SetDefault (“ns3::YansWifiPhy::EnergyDetectionThreshold”,DoubleValue(EnergyDet)); Config::SetDefault (“ns3::YansWifiPhy::CcaMode1Threshold”,DoubleValue(CCAMode1));
if(verbose==1){
LogComponentEnable(“VideoStreaming”, LogLevel (LOG_LEVEL_ALL | LOG_LEVEL_DEBUG | LOG_LEVEL_INFO | LOG_PREFIX_TIME | LOG_PREFIX_NODE| LOG_PREFIX_FUNC));
LogComponentEnable(“VideoPushApplication”, LogLevel (LOG_LEVEL_ALL |LOG_LEVEL_DEBUG | LOG_LEVEL_INFO | LOG_PREFIX_TIME | LOG_PREFIX_NODE| LOG_PREFIX_FUNC));
LogComponentEnable(“AodvRoutingTable”, LogLevel (LOG_LEVEL_ALL |LOG_LEVEL_DEBUG | LOG_LEVEL_INFO | LOG_PREFIX_TIME | LOG_PREFIX_NODE| LOG_PREFIX_FUNC));
LogComponentEnable(“AodvNeighbors”, LogLevel (LOG_LEVEL_ALL |LOG_LEVEL_DEBUG | LOG_LEVEL_INFO | LOG_PREFIX_TIME | LOG_PREFIX_NODE| LOG_PREFIX_FUNC));
LogComponentEnable(“AodvRoutingProtocol”, LogLevel (LOG_LEVEL_ALL |LOG_LEVEL_DEBUG | LOG_LEVEL_INFO | LOG_PREFIX_TIME | LOG_PREFIX_NODE| LOG_PREFIX_FUNC));
Config::ConnectWithoutContext(“/NodeList/*/DeviceList/*/$ns3::WifiNetDevice/Phy/$ns3::YansWifiPhy/PhyTxDrop”,MakeCallback (&PhyTxDrop));
Config::Connect (“/NodeList/*/DeviceList/*/Mac/MacTx”, MakeCallback (&DevTxTrace));
Config::Connect (“/NodeList/*/DeviceList/*/Mac/MacRx”, MakeCallback (&DevRxTrace));
Config::Connect (“/NodeList/*/DeviceList/*/Phy/State/RxOk”, MakeCallback (&PhyRxOkTrace));
Config::Connect (“/NodeList/*/DeviceList/*/Phy/State/RxError”, MakeCallback (&PhyRxErrorTrace));
Config::Connect (“/NodeList/*/DeviceList/*/Phy/State/Tx”, MakeCallback (&PhyTxTrace));
Config::Connect (“/NodeList/*/DeviceList/*/Phy/State/State”, MakeCallback (&PhyStateTrace));
Config::Connect (“/NodeList/*/$ns3::ArpL3Protocol/Drop”, MakeCallback (&ArpDiscard));
Config::Connect (“/NodeList/*/$ns3::aodv::RoutingProtocol/ControlMessageTrafficSent”, MakeCallback (&GenericPacketTrace));
Config::Connect (“/NodeList/*/$ns3::aodv::RoutingProtocol/ControlMessageTrafficReceived”, MakeCallback (&GenericPacketTrace));
}
Current Work progress
NS3 Projects for MS Students
NS3 Projects for B.E,B.Tech students
NS3 Projects for M.E,M.Tech Students
NS3 Projects for PhD Scholars
We assist PhD Scholars in Publishing Papers in Reputed journals for NS3 Simulator Projects.
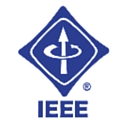

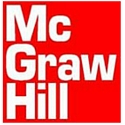
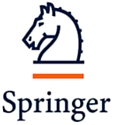

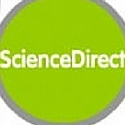



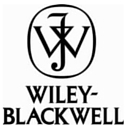

