NS3 VERTICAL HANDOVER PROJECTS provide mobile users to switch among the networks with different technologies like WiFI to WiMax. So in heterogeneous networks VHO is mainly used to carry NS3 Vertical handover projects.
Types of decision schemes in vertical handover:
- Trusted Distributed Vertical Handover Decision (T-DVHD).
- Distributed Vertical Handover Decision (D-VHD).
- Centralized Vertical Handover Decision (C-VHD).
Different phases of ns3 vertical handover projects:
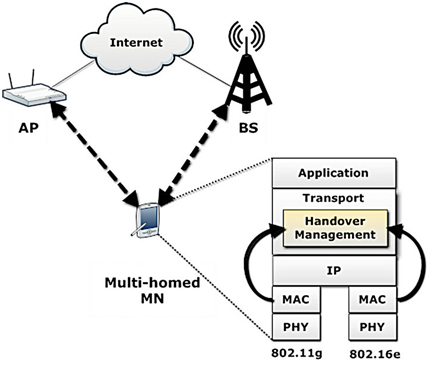
Sample code for ns3 vertical handover projects :
int main (int argc, char *argv[])
{
uint16_t numberOfUes = 1;
uint16_t numberOfEnbs = 2;
uint16_t numBearersPerUe = 2;
double simTime = 0.300;
double distance = 100.0;
Config::SetDefault (“ns3::UdpClient::Interval”, TimeValue (MilliSeconds (10)));
Config::SetDefault (“ns3::UdpClient::MaxPackets”, UintegerValue (1000000));
Config::SetDefault (“ns3::LteHelper::UseIdealRrc”, BooleanValue (false));
CommandLine cmd;
cmd.AddValue (“numberOfUes”, “Number of UEs”, numberOfUes);
cmd.AddValue (“numberOfEnbs”, “Number of eNodeBs”, numberOfEnbs);
cmd.AddValue (“simTime”, “Total duration of the simulation (in seconds)”, simTime);
cmd.Parse (argc, argv);
Ptr lteHelper = CreateObject ();
Ptr epcHelper = CreateObject ();
lteHelper->SetEpcHelper (epcHelper);
lteHelper->SetSchedulerType (“ns3::RrFfMacScheduler”);
lteHelper->SetHandoverAlgorithmType (“ns3::NoOpHandoverAlgorithm”); // disable automatic handover
Ptr pgw = epcHelper->GetPgwNode ();
NodeContainer remoteHostContainer;
remoteHostContainer.Create (1);
Ptr remoteHost = remoteHostContainer.Get (0);
InternetStackHelper internet;
internet.Install (remoteHostContainer);
PointToPointHelper p2ph;
p2ph.SetDeviceAttribute (“DataRate”, DataRateValue (DataRate (“100Gb/s”)));
p2ph.SetDeviceAttribute (“Mtu”, UintegerValue (1500));
p2ph.SetChannelAttribute (“Delay”, TimeValue (Seconds (0.010)));
NetDeviceContainer internetDevices = p2ph.Install (pgw, remoteHost);
Ipv4AddressHelper ipv4h;
ipv4h.SetBase (“1.0.0.0”, “255.0.0.0”);
Ipv4InterfaceContainer internetIpIfaces = ipv4h.Assign (internetDevices);
Ipv4Address remoteHostAddr = internetIpIfaces.GetAddress (1);
Ipv4StaticRoutingHelper ipv4RoutingHelper;
Ptr remoteHostStaticRouting = ipv4RoutingHelper.GetStaticRouting (remoteHost->GetObject ());
remoteHostStaticRouting->AddNetworkRouteTo (Ipv4Address (“7.0.0.0”), Ipv4Mask (“255.0.0.0”), 1);
NodeContainer ueNodes;
NodeContainer enbNodes;
enbNodes.Create (numberOfEnbs);
ueNodes.Create (numberOfUes);
Ptr positionAlloc = CreateObject ();
for (uint16_t i = 0; i < numberOfEnbs; i++) { positionAlloc->Add (Vector (distance * 2 * i – distance, 0, 0));
}
for (uint16_t i = 0; i < numberOfUes; i++) { positionAlloc->Add (Vector (0, 0, 0));
}
MobilityHelper mobility;
mobility.SetMobilityModel (“ns3::ConstantPositionMobilityModel”);
mobility.SetPositionAllocator (positionAlloc);
mobility.Install (enbNodes);
mobility.Install (ueNodes);
NetDeviceContainer enbLteDevs = lteHelper->InstallEnbDevice (enbNodes);
NetDeviceContainer ueLteDevs = lteHelper->InstallUeDevice (ueNodes);
internet.Install (ueNodes);
Ipv4InterfaceContainer ueIpIfaces;
ueIpIfaces = epcHelper->AssignUeIpv4Address (NetDeviceContainer (ueLteDevs));
for (uint32_t u = 0; u < ueNodes.GetN (); ++u)
{
Ptr ueNode = ueNodes.Get (u);
Ptr ueStaticRouting = ipv4RoutingHelper.GetStaticRouting (ueNode->GetObject ());
ueStaticRouting->SetDefaultRoute (epcHelper->GetUeDefaultGatewayAddress (), 1);
}
for (uint16_t i = 0; i < numberOfUes; i++) { lteHelper->Attach (ueLteDevs.Get (i), enbLteDevs.Get (0));
}
NS_LOG_LOGIC (“setting up applications”);
uint16_t dlPort = 10000;
uint16_t ulPort = 20000;
Ptr startTimeSeconds = CreateObject ();
startTimeSeconds->SetAttribute (“Min”, DoubleValue (0));
startTimeSeconds->SetAttribute (“Max”, DoubleValue (0.010));
for (uint32_t u = 0; u < numberOfUes; ++u)
{
Ptr ue = ueNodes.Get (u);
Ptr ueStaticRouting = ipv4RoutingHelper.GetStaticRouting (ue->GetObject ());
ueStaticRouting->SetDefaultRoute (epcHelper->GetUeDefaultGatewayAddress (), 1);
for (uint32_t b = 0; b < numBearersPerUe; ++b)
{
++dlPort;
++ulPort;
ApplicationContainer clientApps;
ApplicationContainer serverApps;
NS_LOG_LOGIC (“installing UDP DL app for UE ” << u);
UdpClientHelper dlClientHelper (ueIpIfaces.GetAddress (u), dlPort);
clientApps.Add (dlClientHelper.Install (remoteHost));
PacketSinkHelper dlPacketSinkHelper (“ns3::UdpSocketFactory”,
InetSocketAddress (Ipv4Address::GetAny (), dlPort));
serverApps.Add (dlPacketSinkHelper.Install (ue));
NS_LOG_LOGIC (“installing UDP UL app for UE ” << u);
UdpClientHelper ulClientHelper (remoteHostAddr, ulPort);
clientApps.Add (ulClientHelper.Install (ue));
PacketSinkHelper ulPacketSinkHelper (“ns3::UdpSocketFactory”,
InetSocketAddress (Ipv4Address::GetAny (), ulPort));
serverApps.Add (ulPacketSinkHelper.Install (remoteHost));
Ptr tft = Create ();
EpcTft::PacketFilter dlpf;
dlpf.localPortStart = dlPort;
dlpf.localPortEnd = dlPort;
tft->Add (dlpf);
EpcTft::PacketFilter ulpf;
ulpf.remotePortStart = ulPort;
ulpf.remotePortEnd = ulPort;
tft->Add (ulpf);
EpsBearer bearer (EpsBearer::NGBR_VIDEO_TCP_DEFAULT);
lteHelper->ActivateDedicatedEpsBearer (ueLteDevs.Get (u), bearer, tft);
Time startTime = Seconds (startTimeSeconds->GetValue ());
serverApps.Start (startTime);
clientApps.Start (startTime);
} }
lteHelper->AddX2Interface (enbNodes);
lteHelper->HandoverRequest (Seconds (0.100), ueLteDevs.Get (0), enbLteDevs.Get (0), enbLteDevs.Get (1));
lteHelper->EnablePhyTraces ();
lteHelper->EnableMacTraces ();
lteHelper->EnableRlcTraces ();
lteHelper->EnablePdcpTraces ();
Ptr rlcStats = lteHelper->GetRlcStats ();
rlcStats->SetAttribute (“EpochDuration”, TimeValue (Seconds (0.05)));
Ptr pdcpStats = lteHelper->GetPdcpStats ();
pdcpStats->SetAttribute (“EpochDuration”, TimeValue (Seconds (0.05)));
Config::Connect (“/NodeList/*/DeviceList/*/LteEnbRrc/ConnectionEstablished”,
MakeCallback (&NotifyConnectionEstablishedEnb));
Config::Connect (“/NodeList/*/DeviceList/*/LteUeRrc/ConnectionEstablished”,
MakeCallback (&NotifyConnectionEstablishedUe));
Config::Connect (“/NodeList/*/DeviceList/*/LteEnbRrc/HandoverStart”,
MakeCallback (&NotifyHandoverStartEnb));
Config::Connect (“/NodeList/*/DeviceList/*/LteUeRrc/HandoverStart”,
MakeCallback (&NotifyHandoverStartUe));
Config::Connect (“/NodeList/*/DeviceList/*/LteEnbRrc/HandoverEndOk”,
MakeCallback (&NotifyHandoverEndOkEnb));
Config::Connect (“/NodeList/*/DeviceList/*/LteUeRrc/HandoverEndOk”,
MakeCallback (&NotifyHandoverEndOkUe));
Simulator::Stop (Seconds (simTime));
Simulator::Run ();
Simulator::Destroy ();
return 0;
}
Current Work progress
NS3 Projects for MS Students
NS3 Projects for B.E,B.Tech students
NS3 Projects for M.E,M.Tech Students
NS3 Projects for PhD Scholars
We assist PhD Scholars in Publishing Papers in Reputed journals for NS3 Simulator Projects.
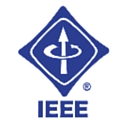

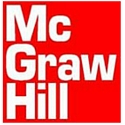
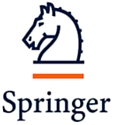

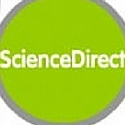



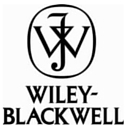

